What is Courtside
Courtside is a mobile app that allows users to fully customize their sports experience by following the exact information they want to see. Users can follow their favorite sports, teams, players, and even individual stats.
The app is built with React Native (using the Expo platform) and Typescript on the frontend. On the backend, the Flask framework serves as our API and MongoDB is used for our database.
What is Courtside (Currently)
Users can follow their favorite sports Basketball, teams, players, and even individual stats. User data is saved on the server, so they can access Courtside from any device.
The server is currently deployed on a service called PythonAnywhere .
This Week
This week was the conclusion of the fifth project sprint, so the main focus of this week was wrapping up the required deliverables for the sprint and preparing our presentation. The main deliverable of this sprint were unit tests for our app.
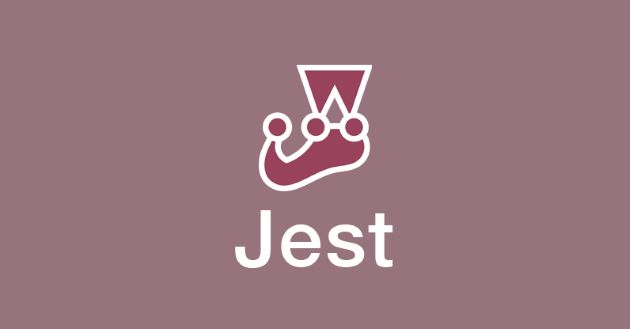
Jest Logo
So after setting up the Jest test runner last week this week my main focus was on creating unit tests for the components on the frontend.
Testing 1 2 3, Testing 1 2 3
Our app has many atomic components. These components are things like different kinds of buttons or inputs, which are used in many places throughout the app. These components were the main focus of my unit testing.
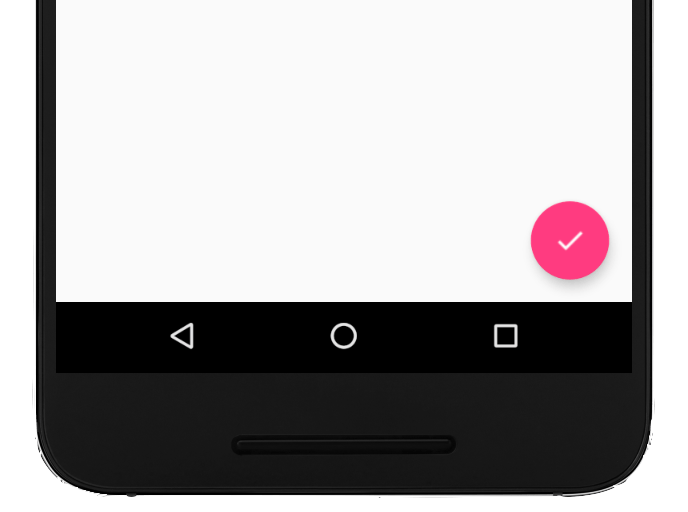
FAB Example from Android Developer docs
For instance, we have a component called a FAB, which is a Floating Action Button. They are a common component in mobile app, and we use them on a few of our screens.
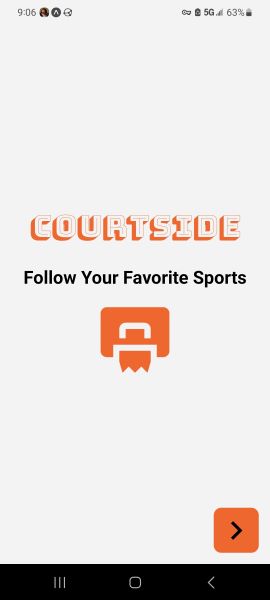
The FAB on our Sport Selection Screen
For our FAB, the developer can specify a few things about it:
- The content inside the button
- The position of the button (the left or right corner)
- The background color of the button
- The function that runs when the button is pressed
These props—as they’re called—are a great blueprint for what we can test about this component.
For instance, the following test checks to see if we can successfully set the background color:
it('has the provided color as its background color', () => {
render(
<FAB onPress={jest.fn()} color={ORANGE}>
<Text>text</Text>
</FAB>
);
const btn = screen.getByTestId('FAB');
expect(btn).toHaveStyle({ backgroundColor: ORANGE });
});
A quick breakdown of that code:
- Line 1 names the test
- Lines 2-5 render the component and initialize its testing state (the background color is being set here)
- Line 8 gets the FAB in a variable by looking for its ID
- Line 10 checks to see if the style in applied to the button
This test checks to see if the function is called when the button is pressed.
it('calls the onPress function when clicked', () => {
const mockFn = jest.fn();
render(
<FAB onPress={mockFn} color={ORANGE}>
<Text>text</Text>
</FAB>
);
const btn = screen.getByTestId('FAB');
fireEvent.press(btn);
expect(mockFn).toHaveBeenCalledTimes(1);
});
The Breakdown:
- Line 2 here creates a fake function that we can pass into the component and test later on.
- Line 11 simulates a button click
- Line 13 checks to see that the fake function has only been called once
Finally, this test makes sure that an icon can be rendered as a child component.
it('renders icon as button child', async () => {
render(
<FAB onPress={jest.fn()} color={ORANGE}>
<MaterialIcons
name="search"
testID="icon"
size={40}
color="black"
/>
</FAB>
);
await waitFor(() =>
expect(screen.getByTestId('icon')).toBeOnTheScreen()
);
});
The Breakdown
- Lines 4-9 create an icon component
- Lines 13–15 checks that the icon appears on the screen (we have to
waitFor
it because images take a moment to load)
Beyond this, I wrote 5 other basic tests for the FAB component and many other similar tests for the other components on our frontend, including the ToggleButton
ErrorBox
, EmailInput
, and others.
Problems
Not too many issues this week. Of course, I wish I could have done things besides pumping out unit tests, but that had to be done this sprint. We also had a big focus on the presentation and preparing demos, which also crunched down dev time.
Next Week
Overarching Goals
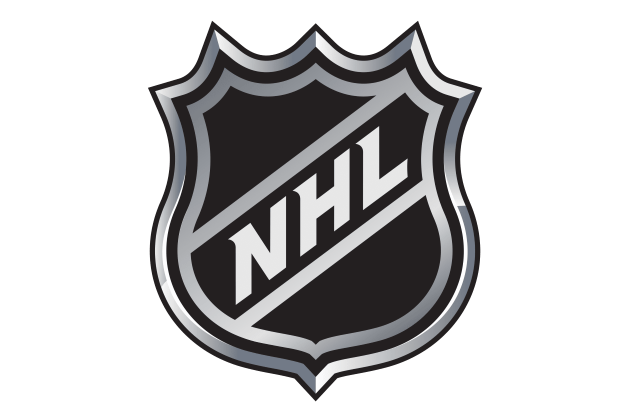
NHL Logo
This next sprint is going to be very exciting because we are finally working to bring a new sport into Courtside: the NHL. So my main job the next few weeks is going to be drawing up plans to generalize the frontend components and backend routes to accommodate new sports. I’m excepting to be working a lot with the Flask API (creating new routes) as well as React Query (to bring the data into the frontend) these next few weeks.
Pet Project
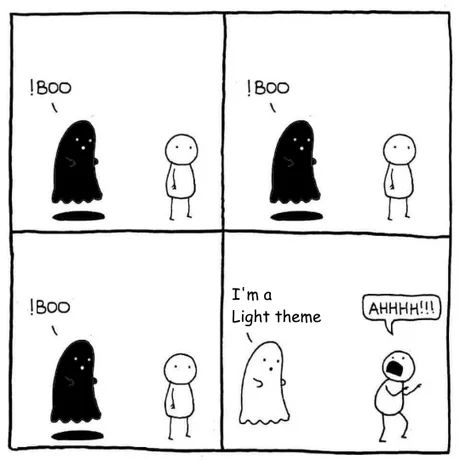
Dark Mode meme
This next sprint, my pet experiment is going to be adding dark mode to the app (so we can be a proper tech company.) I’ve looked into the React Native Appearance API and the theming system within React Navigation, which both seem like promising leads to add this nice-to-have feature!