Hello, my name is Benji Riethmeier, and I am a full stack engineer for the Courtside project, which is being done for my Computer Science Senior Project at the University of Tulsa.
What is Courtside
Courtside is a mobile app that allows users to fully customize their sports experience by following the exact information they want to see. Users can follow their favorite sports, teams, players, and even individual stats.
The app is built with React Native (using the Expo platform) and Typescript on the frontend. On the backend, the Flask framework serves as our API and MongoDB is used for our database. For
This Week
Tasks
The current unit of our senior software class is focused on testing our applications, so we were required to start writing unit tests for the frontend. It was my task to set up a test runner for unit testing and integration testing the frontend.
The other main task was allowing users to set up certain parts of their account while they are creating it. This includes allowing users to choose sports to follow and teams to follow while registering an account.
Testing
For unit testing, I decided to use Jest since it is well integrated with React, React Native, and Expo. In addition, React Native Testing Library was added to allow us to create tests that are easier to maintain and are more reliable. This is done by providing functions that wrap functionality in the bare-bones React test renderer, which also enforce best testing practices. This library also provides more ways to match and find React Native elements and components.
I also wrote some basic tests to demonstrate for the team how to write unit tests in Jest. As an example, I used the DangerButton component, which shows an alert after pushing the button to ensure the user wants to do the button’s action.
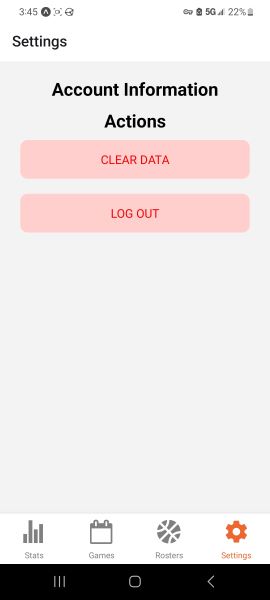
Two DangerButtons in the app
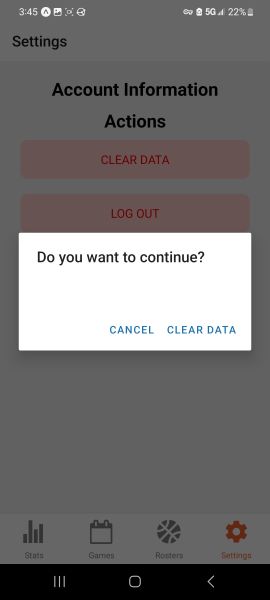
The alert from pushing the Clear Data button
describe("Testing Danger Button Component", () => {
beforeEach(() => jest.resetAllMocks());
it("Displays the button text", () => {
render(<DangerButton text="Button Text" onPress={jest.fn()} />)
const text = screen.getByText('Button Text');
expect(text).toBeOnTheScreen();
});
it("Shows an alert when pressed", () => {
const mockFn = jest.fn();
jest.spyOn(Alert, 'alert');
render(<DangerButton text="test" onPress={mockFn} />);
fireEvent.press(screen.getByText('test'));
expect(Alert.alert).toHaveBeenCalled();
});
})
The tests check to make sure the button displays the given text, that it shows an alert when pressed, and that it doesn’t show an alert when pressed and disabled.
Account Setup
The user can now set up parts of their account while they are creating an account. This required me to create two new screens: a screen that allows users to select what sports they want to follow (Basketball is still the only option) and a screen that allows users to follow teams. The team following screen for setup had to be different from the team following screen within the main navigation due to how the data has to be packaged and processed after the user makes their selection.
The team following screen was also updated to have a search bar like the stat selection screen.
After picking their initial preferences, the user is sent to the sign-up screen that we have had all along. The initial preferences are sent to the Flask API with the HTTP request that creates an account. This required some small changes to be made to the /users/signUp
route (i.e., sending more information in the request body.)
Now, after the user verifies their email, their initial team preferences will be in the app.
Problems
The biggest issue this week was getting an integration testing framework into the app. I tried setting up Detox to run integration tests, but this solution does not work particularly well with an Expo workflow. There are community driven efforts to allow Detox to work with Expo, but getting it set up would require lots of extra configurations and installing multiple versions of different packages to get it to work.
So far there does not seem to be an easy solution to this, but I will keep on looking for something that will work with Expo.
Next Week
This next week, I want to continue working to see if I can find an integration testing framework for React Native and Expo (since Jest is not well suited for the task.)
I also want to start work on integrating more sports into the app. Jake found an API that would let us bring NHL data into the app, so I’m excited to start working with him and the backend team to add that data into the app. This will not be a one-week project as many of our components and routes will have to be refactored to be more general (to allow for different sports), but I’m excited to start!